Generic Messenger
Available since 1.26.0
Set Up a Generic Messenger
Currently, built-in messengers are only provided to send messages via Twilio or email. If you need to connect to a messaging provider other than Twilio, whether you want to send SMS messages or use a different transport layer such as push notifications, you can use the Generic messenger configuration.
The generic messenger allows for a simple JSON REST API integration which allows you to receive a message from FusionAuth to an arbitrary URL. The Generic Message Receiver at this URL may then make an API call to any third party messaging provider.
To create a new generic messenger, navigate to Settings -> Messengers . Click Add Generic Messenger from the dropdown in the upper right.
Add Generic Messenger
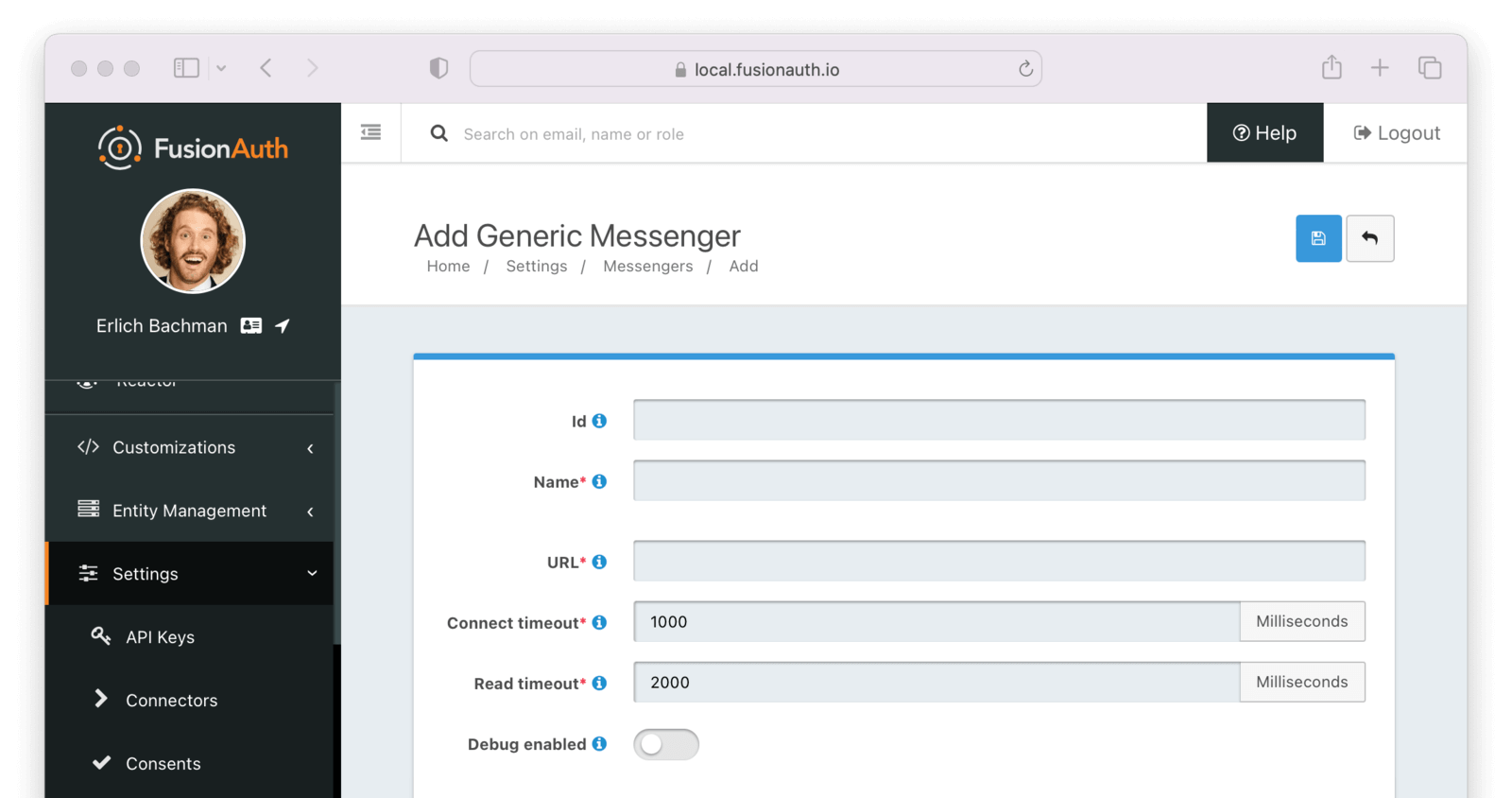
Complete the following fields:
Form Fields
Id
A unique UUID is auto generated if left blank.
Name
requiredFor display only. Name of this generic messenger.
URL
requiredThis is the URL of the messaging service you wish to connect to.
Connect timeout
requiredIn milliseconds, how long to keep the socket open when connecting to the messenger. Must be an integer and greater than 0.
Read timeout
requiredIn milliseconds, how long to keep the socket open when reading to the messenger. Must be an integer and greater than 0.
Debug enabled
When enabled, each message sent using this messenger will generate a new Debug Event Log which can be viewed using the Event Log API or from the admin UI by navigating to System -> Event Log .
Security
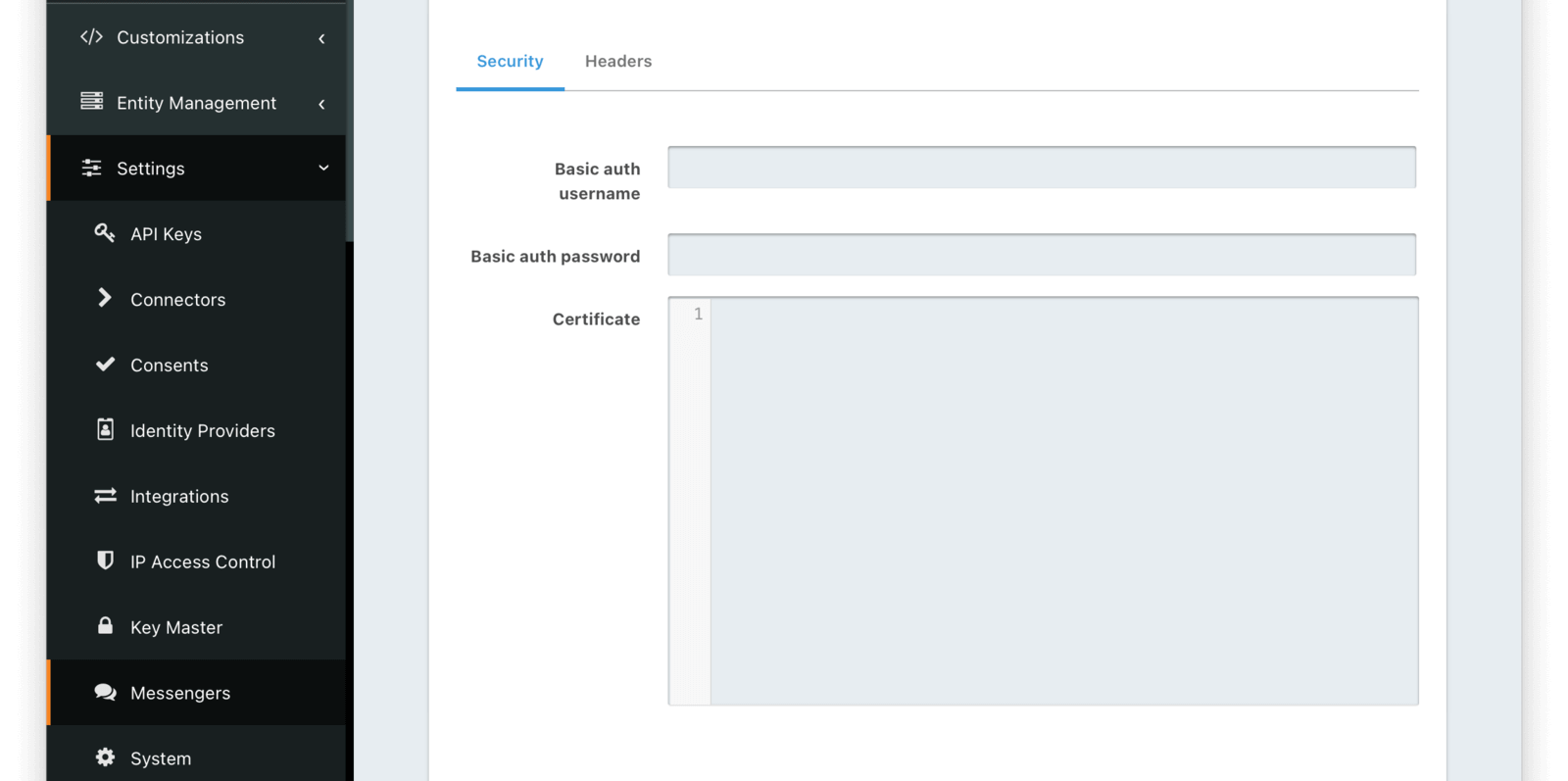
Form Fields
Basic auth username
Username used to authenticate with the generic messenger
Basic auth password
Password used to authenticate with the generic messenger
Certificate
The SSL certificate in PEM format to be used when connecting to the messenger API endpoint. When provided an in memory keystore will be generated in order to complete the HTTPS connection to the messenger API endpoint.
Headers
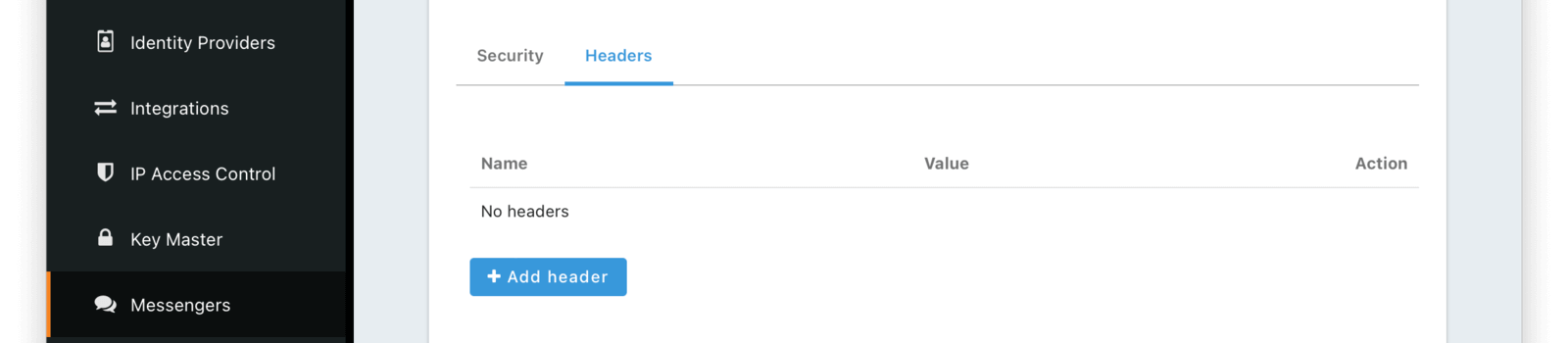
Form Fields
Add Headers
In these fields, you can add custom key and value pairs. These will be sent as headers on the request.
Testing Your Configuration
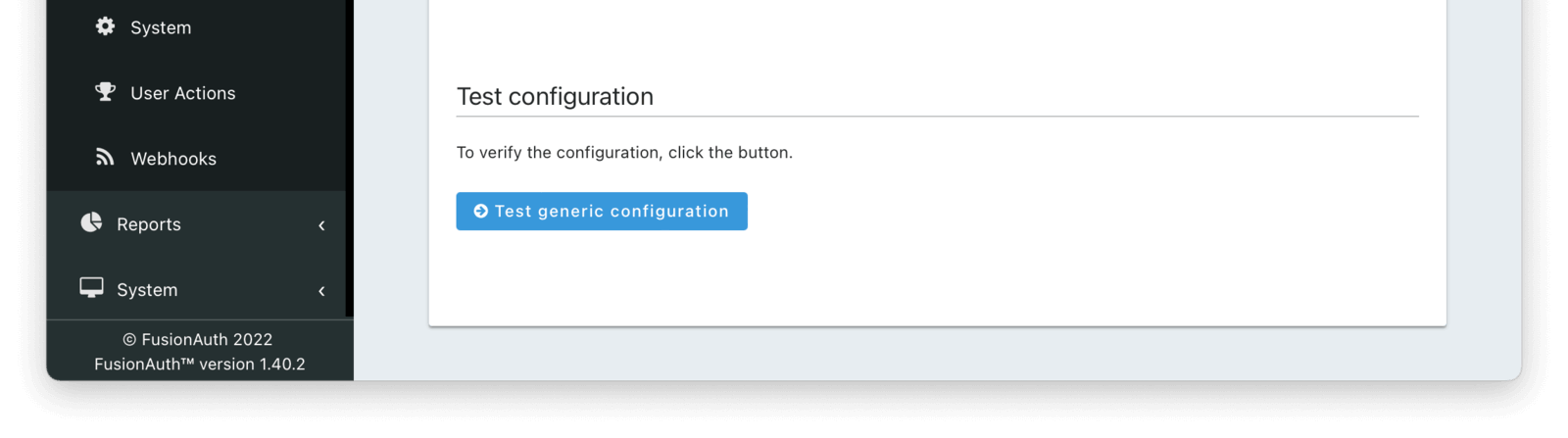
You also can test your generic messenger configuration. By hitting Test generic configuration
FusionAuth will fire a JSON message to the messenger to ensure everything is set up correctly.
Writing the Generic Message Receiver
You need to write a server side component, a receiver, which will be deployed at a URL to consume the message sent from FusionAuth. At that point, it can proxy the request to any third party messaging service. In other words, a FusionAuth Generic Messenger is a thin coordination layer between FusionAuth and other messaging services. An application with the proper permissions, code and configuration to relay a message must exist at the configured URL. This receiver component may be written in any language that can consume a JSON message.
The request to your endpoint will be delivered as JSON. When your application receives this message from FusionAuth, it should take whatever steps necessary to send the message, such as:
- call into a SDK provided by the third party
- make an API request to a vendor provided endpoint
- call multiple internal APIs
- anything else that may be required
Request
phoneNumber
StringThe phone number of the user to which this message should be sent.
textMessage
StringThe message text to send. This is built from the configured message template and is localized.
type
StringThe type of the message. This will always be SMS
.
Example Message Payload JSON
{
"phoneNumber": "3035551212",
"textMessage": "Two Factor Code: 111111",
"type": "SMS"
}
Response
If the message was processed successfully, return a status code in the 200
to 299
range. No further processing will be performed by FusionAuth.
If the receiver is unsuccessful at sending the message, for whatever reason, return a status code outside of that range.
Securing the Generic Message Receiver
TLS v1.2
The first step in securing your Generic Message Receivers is to ensure that they are using TLS v1.2 or higher. You should be using a web server that is configured with a certificate from a valid certificate authority and to only receive traffic from a secure connection. We also recommend that you disable all older secure protocols including SSL, TLS 1.0 and TLS 1.1.
If you need a certificate, most cloud providers offer them or you can use LetsEncrypt to generate a certificate and ensure it is always up-to-date.
Headers
When you configure your Generic Message Receiver with FusionAuth, you should include a security header of some kind. There are two ways to define a security header:
- Add a Basic Authentication username and password under Security
- Define an HTTP header under the Headers tab
In either case, your Generic Message Receiver code should validate the security header to ensure the request is coming from FusionAuth. Here’s some example code that validates an Authorization
header:
Example Generic Message Receiver
router.route('/fusionauth-generic-messenger').post((req, res) => {
const authorization = req.header('Authorization');
if (authorization !== 'API-KEY') {
res.status(401).send({
'errors': [{
'code': '[notAuthorized]'
}]
});
} else {
// process the request
}
});
Certificates
You may provide an X.509 certificate to use with your Generic Message Receiver. This must be an SSL certificate in PEM format. It is used to establish a TLS connection to the Generic Message Receiver endpoint. Use this option if FusionAuth cannot connect to your Generic Message Receiver without the certificate.
Providing this certificate will build a custom SSL context for requests made for the Generic Message Receiver. Therefore, any other JDK keystores and certificate authority chains will be bypassed for this request.
Firewalls
In addition to using TLS and a security header, you might also want to put a firewall in front of your Generic Message Receiver. In most cases, this firewall will only allow traffic to your Generic Message Receiver that originated from your FusionAuth instance. Depending on how you are hosting your Generic Message Receiver, you might be able to lock down the URL for your Generic Message Receiver specifically. You might also leverage an API gateway or a proxy to ensure that only traffic coming from FusionAuth is routed to your Generic Message Receiver. The exact specifics of deploying and configuring a Firewall are outside the scope of this document, but you can consult the documentation for your proxy, API Gateway or hosting provider to determine how to manage it.
As an example, you can configure an AWS Application Load Balancer so that traffic coming from the IP address of your FusionAuth servers with a URL of https://apis.mycompany.com/fusionauth-generic-messenger
is routed through. You can then configure the Application Load Balancer so that all other traffic to that URL is rejected.
Controlling Traffic with a Proxy
Since version 1.31.0
, FusionAuth can be configured to send all outbound HTTP traffic, including that from a Generic Message Receiver, through a proxy. This allows you examine any messages sent from FusionAuth. You can audit them, only allow connections to certain hosts, or otherwise modify them.
To configure a proxy for FusionAuth outbound traffic, use the proxy.*
configuration options.