JSON Web Tokens and the Login API
Overview
JSON Web Tokens (or JWT for short - pronounced “jot”) is a standard defined as RFC 7519 that provides a portable unit of identity. FusionAuth implements the JWT specification and can provide JWTs as part of the authentication workflows.
If you are using OAuth grants for authentication, only the JWT signing configuration documentation applies.
Much of this tutorial is aimed at those using the Login API, not the hosted login pages.
For more on the OAuth grants and the tokens returned when using them, visit the OAuth documentation.
After a User is authenticated via the Login API or OAuth, FusionAuth creates a JWT and returns it to the caller. This JWT will be cryptographically signed to allow other applications to verify that it was created by FusionAuth.
Configuring JWTs in FusionAuth
FusionAuth provides the ability to configure a couple of aspects of its JWT handling. By navigating to Settings -> Tenants in FusionAuth and selecting the JWT tab, you will see the tenant JWT configuration settings.
The following is an example screenshot of the tenant JWT configuration.
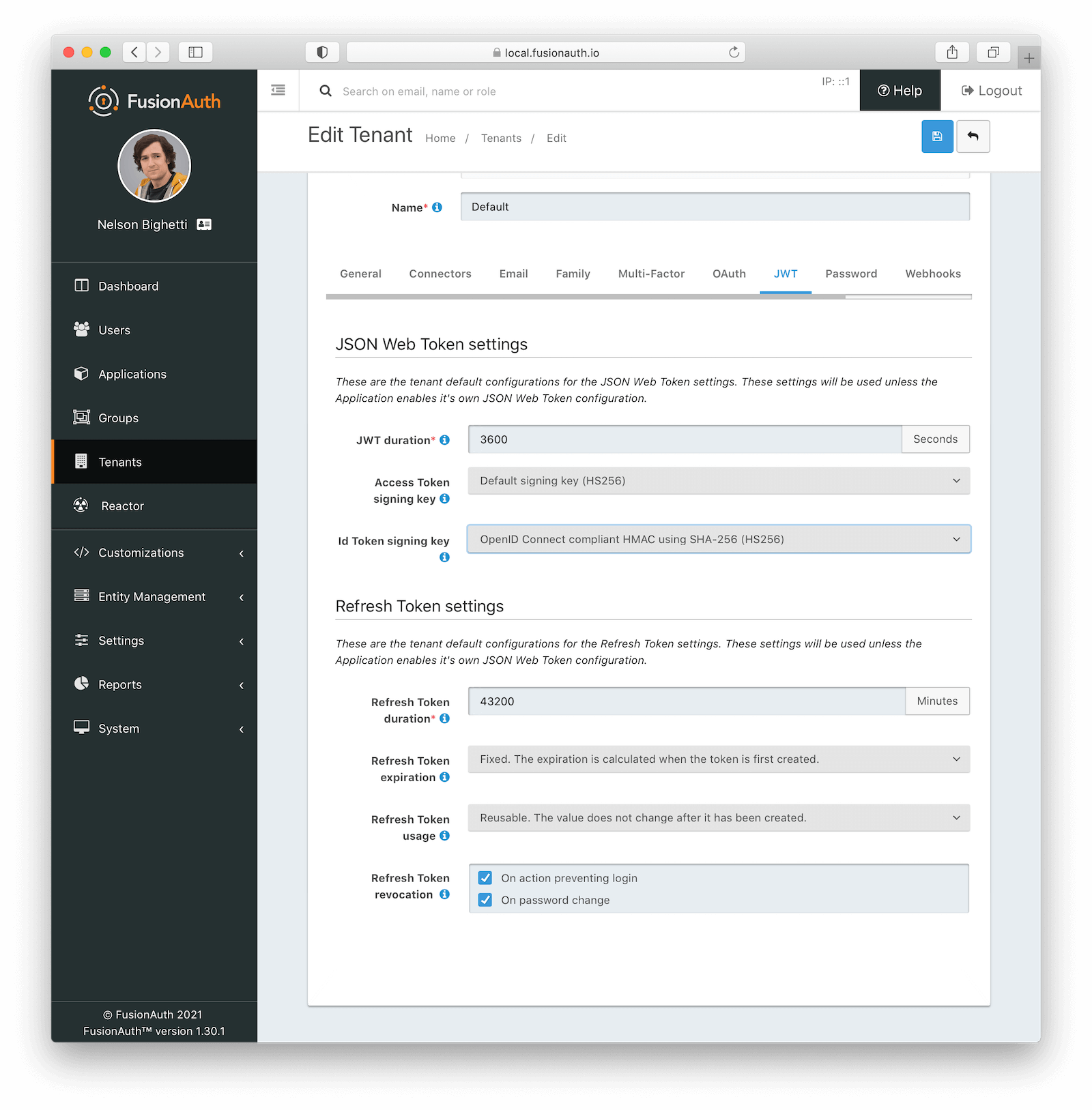
JSON Web Token Settings
JWT duration
requiredThe length of time the issued token (access token and Id token) is valid. JWT tokens are typically short lived.
Access token signing key
The key used to sign the access token JWT.
Id token signing key
The key used to sign the Id token JWT.
Refresh Token Settings
Refresh token duration
requiredDefaults to 43,200The length of time the refresh token is valid. Refresh tokens are typically long lived.
Refresh token expiration
Defaults to FixedThe Refresh token expiration may be either a fixed window, sliding window, or sliding window with a maximum lifetime. By default, the expiration of a refresh token is a fixed length of time from when it was originally issued. With a sliding window expiration, the expiration is calculated from the last time the refresh token was used. However with a sliding window with a maximum lifetime, the expiration is calculated from the last time the refresh token was used until a maximum lifetime value is reached.
For instance, consider the following scenarios given the above configuration. If a refresh token is issued at 1:00pm and has a duration of 60 minutes, if the expiration is fixed, the token will expire at 2:00pm. If, instead, the expiration is a sliding window, then if the refresh token is used at 1:55pm, it would then expire at 2:55pm. If it were then used at 2:50pm, it would expire at 3:50 pm. Lastly if the expiration is a sliding window with a maximum lifetime of 24 hours, then the token could be refreshed every hour (in the same sliding manner as outlined above) up to 23 times. For instance, if a token was issued at 1:55pm on Monday and refreshed every hour, the 23th refresh it would be the last valid refresh request (12:55pm Tuesday would be the last valid refresh event).
Refresh token usage
Defaults to ReusableThe Refresh token usage may be reusable or one time use. By default, a token is reusable and the token does not change after it was issued. With a one time use token, the token value will be changed each time the token is used to refresh a JWT. This means the client must store the new value after each use.
Refresh token revocation
The event or events that will cause refresh tokens to be revoked.
Application Specific Configuration
If you navigate to Applications from the main menu, you can also configure the JWT parameters, including the signing algorithm, on a per application basis. If you don’t select to enable Application specific JWT configuration, the tenant configuration will be used.
The following is an example screenshot of an Application specific JWT configuration.
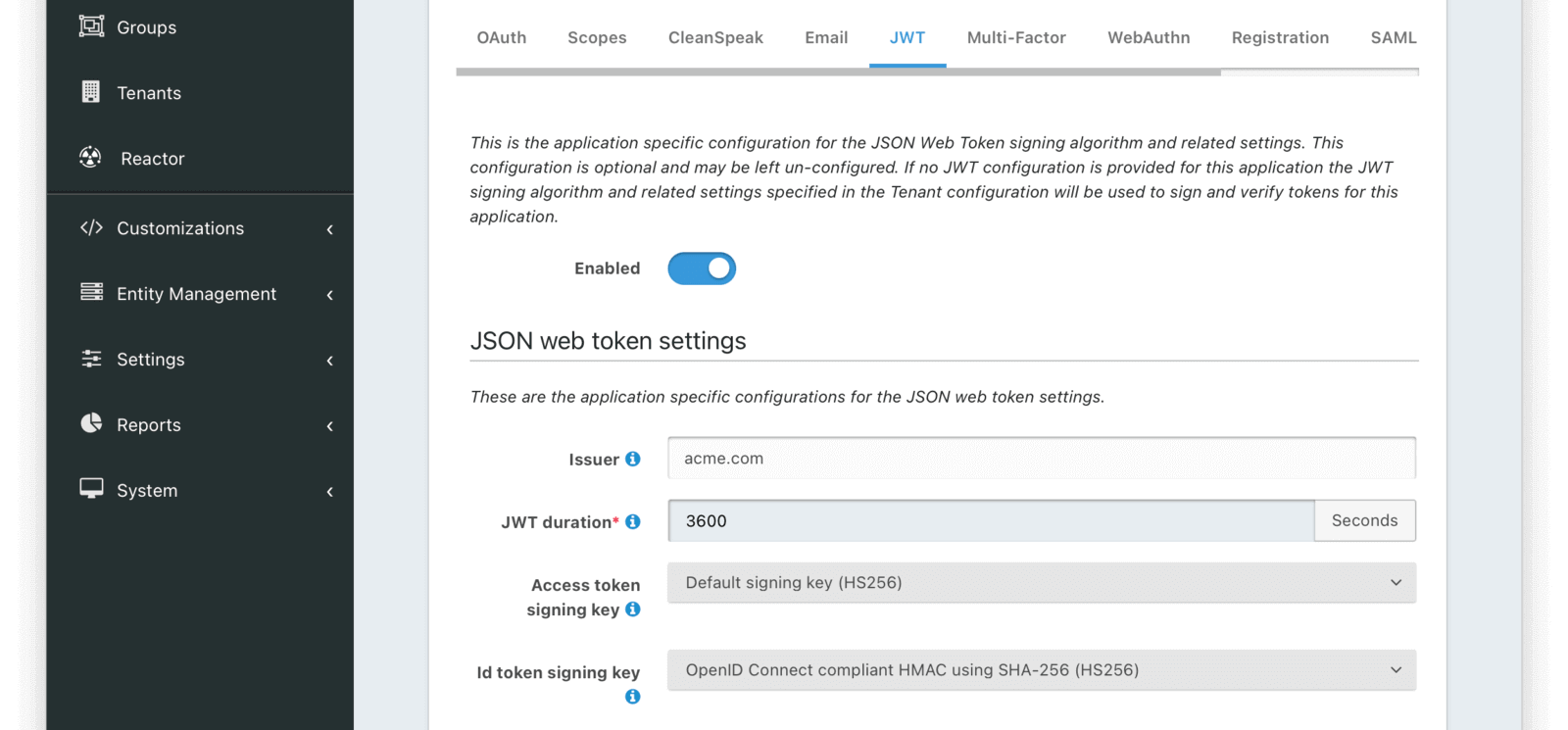
JSON Web Token Settings
Issuer
Read onlyThe issuer used in the iss
claim when building the Access Token and Id Token. This is a read-only value in this configuration. It can be modified in the Tenant configuration.
JWT duration
requiredThe duration in seconds for which a JWT will be valid after creation. After this time has passed the JWT will expire and can no longer be used.
Access token signing key
The signing key used to sign the Access Token (which is a JWT) when a user authentic The signing key used to sign the Access Token (which is a JWT) when a user authenticates against this Application. When this value is not selected, FusionAuth will generate a new key pair and assign it to this configuration.
Id token signing key
The signing key used to sign the Id Token (which is a JWT) when a user authenticates against this Application. When this value is not selected, FusionAuth will generate a new key pair and assign it to this configuration.
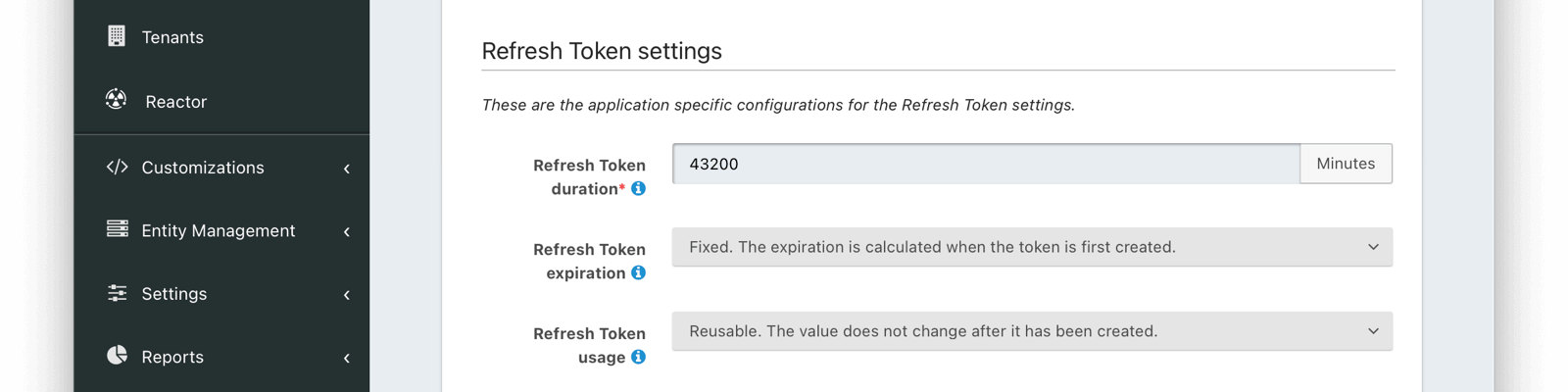
Refresh Token Settings
Refresh token duration
requiredDefaults to 43,200The length of time the refresh token is valid. Refresh tokens are typically long lived.
Refresh token expiration
Defaults to FixedThe Refresh token expiration may be either a fixed window, sliding window, or sliding window with a maximum lifetime. By default, the expiration of a refresh token is a fixed length of time from when it was originally issued. With a sliding window expiration, the expiration is calculated from the last time the refresh token was used. However with a sliding window with a maximum lifetime, the expiration is calculated from the last time the refresh token was used until a maximum lifetime value is reached.
For instance, consider the following scenarios given the above configuration. If a refresh token is issued at 1:00pm and has a duration of 60 minutes, if the expiration is fixed, the token will expire at 2:00pm. If, instead, the expiration is a sliding window, then if the refresh token is used at 1:55pm, it would then expire at 2:55pm. If it were then used at 2:50pm, it would expire at 3:50 pm. Lastly if the expiration is a sliding window with a maximum lifetime of 24 hours, then the token could be refreshed every hour (in the same sliding manner as outlined above) up to 23 times. For instance, if a token was issued at 1:55pm on Monday and refreshed every hour, the 23th refresh it would be the last valid refresh request (12:55pm Tuesday would be the last valid refresh event).
Refresh token usage
Defaults to ReusableThe Refresh token usage may be reusable or one time use. By default, a token is reusable and the token does not change after it was issued. With a one time use token, the token value will be changed each time the token is used to refresh a JWT. This means the client must store the new value after each use.
Lambda Settings
The application specific lambda settings are available even if you choose not to enable additional application specific JWT configuration by leaving the Enable field off.
Access token populate lambda
The lambda to be invoked during the generation of an Access Token (JWT) when a user authenticates against this Application.
Id token populate lambda
The lambda to be invoked during the generation of an Id Token (JWT) when a user authenticates against this Application.
Learn more about the JWT Populate Lambda.
Configuring JWT Signing
FusionAuth provides three configuration locations for JWT signing:
- the tenant default values shown above
- an Application level setting
- with Entity Management, each entity type can have a unique key
FusionAuth supports configurations for HMAC, ECDSA (Elliptic Curve) or RSA based signing algorithms.
Keys are managed in Key Master and can be generated or imported there.
ECDSA & RSA Signing
If you are using FusionAuth in a hybrid environment where applications may be untrusted, asymmetric ECDSA or RSA signing is preferred.
Using this approach allows you to provide applications with a public key to verify the JWT signature while securing the private key in FusionAuth.
Only the party that holds the private key can produce JWTs. It is easier to know for certain who issued and signed the JWT. It will be FusionAuth.
HMAC Signing
If you are in a secure environment and you require better performance, symmetric HMAC signing is best.
The downside of using HMAC signing is that each application requires access to the HMAC secret and you’ll need to ensure it is distributed safely.
Anyone with the secret can both produce a JWT with a valid signature and verify the signature which makes it difficult to know for certain who issued and signed the JWT.
Login and JWTs
When you complete a request to the Login API, FusionAuth will return a JWT in the JSON response body as well as in an HTTP Only session cookie. The cookie has the benefit of allowing web applications to authenticate directly against FusionAuth and managing JWT identities through the browser. The cookie name that the JWT is returned in is called access_token
.
Here is an example of this Set-Cookie
response header that includes a JWT with line breaks and spaces for readability.
Example HTTP Cookie Header
Set-Cookie: access_token=eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.
eyJleHAiOjE0ODUxNDA5ODQsImlhdCI6MTQ4NTEzNzM4NCwiaXNzIjoiYWNtZS5jb20iLCJzdWIiOiIyOWFjMGMxOC0wYjRhLTQyY2YtODJmYy0wM2Q1NzAzMThhMWQiLCJhcHBsaWNhdGlvbklkIjoiNzkxMDM3MzQtOTdhYi00ZDFhLWFmMzctZTAwNmQwNWQyOTUyIiwicm9sZXMiOltdfQ.
Mp0Pcwsz5VECK11Kf2ZZNF_SMKu5CgBeLN9ZOP04kZo;
Secure; HttpOnly
The JSON response body will also contain the JWT in an attribute called token
like this:
Example JSON Response Body with JWT
{
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJleHAiOjE0ODUxNDA5ODQsImlhdCI6MTQ4NTEzNzM4NCwiaXNzIjoiYWNtZS5jb20iLCJzdWIiOiIyOWFjMGMxOC0wYjRhLTQyY2YtODJmYy0wM2Q1NzAzMThhMWQiLCJhcHBsaWNhdGlvbklkIjoiNzkxMDM3MzQtOTdhYi00ZDFhLWFmMzctZTAwNmQwNWQyOTUyIiwicm9sZXMiOltdfQ.Mp0Pcwsz5VECK11Kf2ZZNF_SMKu5CgBeLN9ZOP04kZo",
"user": {
"active": true,
"email": "example@fusionauth.io",
"expiry": 1571786483322,
"id": "00000000-0000-0001-0000-000000000000",
"lastLoginInstant": 1471786483322,
"passwordChangeRequired": false,
"passwordLastUpdateInstant": 1471786483322,
"verified": true
}
}
Skipping JWT Creation
There are some circumstances where you don’t need a JWT returned as part of the Login API response and therefore you can instruct FusionAuth to omit the JWT from the response. This will reduce the latency of calling the Login API because FusionAuth can skip the creation and signing of the JWT. To disable JWTs during authentication, supply the noJWT
parameter in the JSON request body on the Login API.
JWT Payload
FusionAuth provides a few custom claims in addition to some registered claims as defined by RFC 7519 Section 4.1. The following claims will be found in a JWT issued by FusionAuth.
Claims
applicationId
UUIDThe unique Id of the Application for which the User has been authenticated. A JWT can only represent authorization to a single Application.
This claim is only present if the User has a registration to the Application.
To obtain a JWT for another Application you must either authenticate again with a different applicationId
using the Authentication API or utilize the Issue a JWT API to exchange a valid JWT for another.
aud
StringThe audience the JWT is intended for. This registered claim is defined by RFC 7519 Section 4.1.3.
This claim will be equal to the client_id
.
authenticationType
StringThe method used to authenticate the User which resulted in this JWT being generated. The possible values are:
APPLE
- The User was authenticated using Apple. Available since 1.17.0APPLICATION_TOKEN
- The User was authenticated using an Application Authentication Token.EpicGames
-The User was authenticated using Epic Games. Available since 1.28.0FACEBOOK
- The User was authenticated using Facebook. Available since 1.1.0FEDERATED_JWT
- The User was authenticated using a JWT from an external Identity Provider.GENERIC_CONNECTOR
- The user was authenticated using a generic connector. Available since 1.18.0GOOGLE
- The User was authenticated using Google. Available since 1.1.0HYPR
- The User was authenticated using the HYPR provider. Available since 1.12.0JWT_SSO
- A valid JWT authorized to one Application was exchanged for another JWT authorized to a different Application.LDAP_CONNECTOR
- The user was authenticated using an LDAP connector. Available since 1.18.0LINKEDIN
- The user was authenticated using LinkedIn. Available since 1.23.0Nintendo
- The User was authenticated using Nintendo. Available since 1.36.0ONE_TIME_PASSWORD
The User was authenticated using a one time password. Available since 1.5.0OPENID_CONNECT
- The User was authenticated using an external OpenID Connect provider. Available since 1.1.0PASSWORD
- The User was authenticated using a loginId and password combination.PASSWORDLESS
- The user was authenticated using a passwordless login link. Available since 1.5.0PING
- The user was authenticated using aPUT
request on the Login API. This is used to record a login event without prompting for credentials.REFRESH_TOKEN
- The User requested a new JWT using a Refresh Token.REGISTRATION
- The user was created using the Registration API. Available since 1.16.0SAMLv2
- The User was authenticated using an external SAMLv2 provider. Available since 1.6.0SAMLv2IdpInitiated
- The User was authenticated using an external SAMLv2 provider using an IdP Initiated login. Available since 1.28.0SonyPSN
- The User was authenticated using Sony Available since 1.28.0Steam
- The User was authenticated using Steam Available since 1.28.0TWITTER
- The User was authenticated using Twitter. Available since 1.1.0Twitch
- The User was authenticated using Twitch Available since 1.28.0USER_CREATE
- The user was created using the User API. Available since 1.16.0Xbox
- The User was authenticated using Xbox Available since 1.28.0
auth_time
LongAvailable since 1.36.0The time of the initial authentication request, expressed as UNIX time which is the number of seconds since Epoch. This claim will remain the same even when the token has been re-issued through the use of a Refresh Token.
email
StringThe email address of the User whose claims are represented by this JWT.
Removed in 1.50.0In version 1.50.0
and later this claim is not returned when the oauthConfiguration.scopeHandlingPolicy value of the Application is Strict
.
email_verified
BooleanThe OpenId Connect claim indicating if the User’s email has been verified.
Removed in 1.50.0In version 1.50.0
and later this claim is not returned when the oauthConfiguration.scopeHandlingPolicy value of the Application is Strict
.
exp
LongThe expiration instant of the JWT, expressed as UNIX time which is the number of seconds since Epoch. This registered claim is defined by RFC 7519 Section 4.1.4.
iat
LongThe instant that the JWT was issued, expressed as UNIX time which is the number of seconds since Epoch. This registered claim is defined by RFC 7519 Section 4.1.6.
jti
StringAvailable since 1.18.0The unique identifier for this JWT. This registered claim is defined by RFC 7519 Section 4.1.7.
iss
StringThe issuer of the JWT. For FusionAuth, this is always the value defined in the tenant JWT configuration. This registered claim is defined by RFC 7519 Section 4.1.1.
preferred_username
StringAvailable since 1.5.0The username of the User whose claims are represented by this JWT.
Removed in 1.50.0In version 1.50.0
and later this claim is not returned when the oauthConfiguration.scopeHandlingPolicy value of the Application is Strict
.
roles
Array<String>The roles assigned to the User in the authenticated Application. This claim is only present if the User has a registration to the Application.
scope
StringAvailable since 1.50.0The scope of the Access token. This meaning of this field is specified by RFC 6749 Section 3.3.
Contains the validated and consented OAuth scopes from the initial authentication request. See Scopes for more detail on scope consent.
sid
StringAvailable since 1.37.0The unique Id of the refresh token returned along with this access token when the offline_access
scope was requested. This unique Id is the persistent identifier for this refresh token, and will not change even when using one-time use refresh tokens. This value may optionally be used to revoke the token using the Refresh Token API.
sub
UUIDThe subject of the access token. This value is equal to the User’s unique Id in FusionAuth. This registered claim is defined by RFC 7519 Section 4.1.2.
tid
UUIDAvailable since 1.36.0The FusionAuth Tenant unique Id.
Example JWT
Here is an example JWT that might be returned from FusionAuth:
Example JWT
{
"applicationId": "469b0ba1-a849-4603-883e-3b05c0d2b7ce",
"aud": "469b0ba1-a849-4603-883e-3b05c0d2b7ce",
"authenticationType": "PASSWORD",
"email": "richard@fusionauth.io",
"exp": 1504112919754,
"iat": 1504103919754,
"iss": "acme.com",
"roles": [
"role 1",
"role 2"
],
"sub": "6558c73f-b345-4917-9aac-0feab21eeeeb"
}
Refresh Tokens
Refresh tokens are a method of allowing a User to stay logged into an Application for a long period of time without requiring them to type in their password. This method is often used by Applications that don’t store sensitive data such as games and social networks.
To receive a refresh token from the Login API, enable Refresh Tokens for the application. Using the FusionAuth UI, navigate to Settings -> Applications -> Security Tab. Here you will find the Login API settings. Ensure that both Generate Refresh Tokens and Enable JWT refresh fields are enabled.
FusionAuth provides refresh tokens in the response from the Login API provided that you supply these elements:
loginId
- the User’s login Idpassword
- the User’s password or Authentication TokenapplicationId
- the identifier for the Application the user is logging into
If all of these attributes are supplied to the Login API then FusionAuth will produce a refresh token.
If any of the required elements are missing, no refresh token will be generated.
The refresh token will be returned in the JSON in the response body as well as in a cookie header. The refresh token will be in a parameter called refreshToken
in the JSON response and the HTTP Only persistent cookie will be named refresh_token
.
Here is an example of this Set-Cookie
response header for a refresh token.
Example HTTP Cookie Header
Set-Cookie: refresh_token=eu1SsrjsiDf3h3LryUjxHIKTS0yyrbiPcsKF3HDp; Max-Age=2592000; Expires=Fri, 29-Sep-2017 15:20:24 GMT; Secure; HttpOnly
Here is an example of the JSON response body that contains the refresh token:
Example JSON with Refresh Token
{
"refreshToken": "eu1SsrjsiDf3h3LryUjxHIKTS0yyrbiPcsKF3HDp",
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJleHAiOjE1MDQxMDY0ODQsImlhdCI6MTUwNDEwNjQyNCwiaXNzIjoiaW52ZXJzb2Z0LmNvbSIsInN1YiI6ImUzMDIzMmZiLTIxN2EtNDllYi1iN2QxLTI5YzhhNWVmZmM1YiIsImFwcGxpY2F0aW9uSWQiOiIzYzIxOWU1OC1lZDBlLTRiMTgtYWQ0OC1mNGY5Mjc5M2FlMzIiLCJhdXRoZW50aWNhdGlvblR5cGUiOiJQQVNTV09SRCIsInJvbGVzIjpbImFkbWluIl19.eNF4F0iT8qUtyr3kb_-RSM-jibaP0w-419sD94N3Gkk",
"user": {
"active": true,
"email": "example@fusionauth.io",
"expiry": 1571786483322,
"id": "00000000-0000-0001-0000-000000000000",
"lastLoginInstant": 1471786483322,
"passwordChangeRequired": false,
"passwordLastUpdateInstant": 1471786483322,
"verified": true
}
}
Refresh Tokens are considered sensitive information. The token must be secured on the User’s device. If a Refresh Token is compromised, the token bearer may perform authenticated requests on behalf of the User.
Once you have a refresh token on the device, you can call the Refresh a JWT API to get a new JWT from FusionAuth using the refresh token. Using this pattern allows you to perform authenticated actions using the JWT without prompting the User to authenticate as long as the refresh token is active.