Logout And Session Management
Overview
This guide documents logout and session management features. Logout, or sign out, processes revoke users’ access to applications and functionality. Session management controls the session and therefore continued access to application functionality and data.
For web and mobile applications, a session allows servers receiving requests over HTTP to group requests made by a user or application together. OWASP, an open-source security project, defines a session as:
… a sequence of network HTTP request and response transactions associated with the same user. Modern and complex web applications require the retaining of information or status about each user for the duration of multiple requests. Therefore, sessions provide the ability to establish variables – such as access rights and localization settings – which will apply to each and every interaction a user has with the web application for the duration of the session.
Applications which delegate authentication to FusionAuth may delegate session management to FusionAuth.
The ChangeBank Scenario
Let’s look at two applications, ChangeBank and ChangeBank Forum. ChangeBank is the application you can build when working through a quickstart. It lets you make change given an amount of money. ChangeBank Forum is a web forum where people share their favorite stories and advice about coins and how change is made. Both applications delegate authentication to FusionAuth.
This guide will explore sessions and logging out functionality with these two example applications.
Sessions Basics
Every session in FusionAuth is associated with all three of:
- a user (a person)
- an application
- a device or software running on a device
Here are examples that result in different sessions:
- Richard logs in to ChangeBank with the Chrome browser on his Windows PC. He gets session A.
- Richard logs in to ChangeBank with the Edge browser on his Windows PC. He gets session B.
- Richard logs in to the ChangeBank Forum with the Safari browser on his iPhone. He gets session C.
- Malia logs in to the ChangeBank Forum with the Safari browser on her iPhone. She gets session D.
Even though Richard is on one Windows PC, he gets both session A and session B because he used different browsers.
There are three types of sessions relevant to this guide:
- An application session is created after FusionAuth has authenticated a user, the application has completed the token exchange, and the user has been logged in to the application. The application almost always creates a session. How exactly this session works (a cookie, value stored in redis, or a database row) is specific to each web or mobile application.
- A FusionAuth SSO session is created when a user checks Keep me signed in , or when a custom theme hard codes that form value. This session is available only when using the hosted login pages and a browser or webview. It allows for transparent, automatic user authentication when a user moves between different web or mobile applications on the same device.
- A centralized session is a FusionAuth refresh token. It represents an application session that is managed by FusionAuth. This session can be read or revoked using the FusionAuth API. It is created by a user login. Both the hosted login pages or the Login API support this type of session.
When this guide refers to sessions without any of these prefixes, the relevant statement applies to all these types of sessions.
Types Of Session Management
When considering session management with FusionAuth, first consider whether you need a centralized session store. Do you want your FusionAuth instance to keep track of sessions for each application?
With centralized session management, you can:
- capture information about users’ sessions across applications such as lifetime, device, or IP address
- log users out of application using the FusionAuth admin UI or the API
- remove sessions with fine grained control according to business logic you write
- have different session lifetimes for applications, but manage those lifetimes in FusionAuth
The downside of centralized sessions is implementation complexity and more reliance on FusionAuth’s APIs.
The alternative to centralized sessions is application managed sessions. These sessions are ended with Front-Channel Logout rather than the API. With application managed sessions, FusionAuth doesn’t keep track of session lifetime or other characteristics. Instead, FusionAuth relies on the applications to manage each session.
With application managed sessions, you:
- will have no values will appear on the Sessions tab under the user details screen in the admin UI, except perhaps the FusionAuth SSO session
- cannot use the API to view or manage application sessions
- won’t have any control over timeouts; they are managed by each application and will need to be configured there
- cannot revoke application sessions via the API, though you will still be able to request revocation
Choosing A Session Management Approach
Here’s a table outlining major differences between centralized sessions and application managed sessions.
Feature | Centralized Sessions | Application Managed Sessions |
---|---|---|
Level of effort | Medium | Low |
Revoke sessions across all applications in a tenant on logout | Yes | Yes |
Revoke sessions for one application on logout | Yes | Yes |
Revoke sessions for more than one and fewer than all applications on logout | Yes | No |
Fine grained session revocation, including via API | Yes | No |
Precise control of session timeout | Yes | No |
Central view of sessions in FusionAuth | Yes | No |
Call to FusionAuth required each time a user interacts with your application | Yes | No |
Works with FusionAuth SSO, including revocation | Yes | Yes |
Works with non browser based applications, such as APIs | Yes | No |
Session revocation webhooks available | Yes | No |
Can be used without using the hosted login pages | Yes | No |
Let’s examine each of these approaches in more detail.
Centralized Sessions
This section documents how to implement a centralized session store using FusionAuth. Let’s use the example ChangeBank and ChangeBank Forum applications mentioned above.
Implementation Steps
- Make sure to set up the ChangeBank and ChangeBank Forum Applications are correctly configured inside FusionAuth. The Application has the Refresh token checkbox toggled in the Enabled Grants section. The Generate refresh tokens setting must be enabled too.
- Each time you build the authorization URL, your
scope
parameter must include the following scopes:offline_access
, which creates the refresh token andopenid
, which asks for an Id token. The authorization URL will therefore include this string:&scope=openid%20offline_access
, since scopes are separated with a URL encoded space. - In the ChangeBank and ChangeBank Forum applications, create an application session following your web or mobile app framework documentation.
- After the user is logged in, store the refresh token Id in the application session. The Id is available in the
sid
parameter in the Id token, or in the access token. Prefer the refresh token Id instead of the refresh token value, since the Id will not change even if the value of the token itself does. This can happen if you are using one-time refresh tokens.- You could also store the refresh token Id in a HttpOnly cookie instead of in a session.
- Whenever the ChangeBank or ChangeBank Forum application receives a request, call the Retrieve Refresh Tokens API, using the stored refresh token Id, to check the status of the refresh token. You can call the REST API directly or using one of the FusionAuth client libraries. If the refresh token doesn’t exist or is invalid, deny the user access and invalidate the application session.
- When a user logs out from either the ChangeBank or ChangeBank Forum application, revoke the refresh token. Use the Revoke Refresh Tokens API. You can revoke all refresh tokens or just a few. The logic around revocation depends on your business needs. See Flexible Revocation for more details.
You are using the refresh token to tie the ChangeBank and ChangeBank Forum applications to FusionAuth. Your applications are confirming session validity with FusionAuth each time a request is received.
Flow Diagrams Of Common Use Cases
Let’s look at some flow diagrams for these four use cases:
- a user logs into ChangeBank and then visits ChangeBank Forum
- a logged in user interacts with ChangeBank
- a user logs out of ChangeBank, which revokes all refresh tokens for that user
- tries to access ChangeBank Forum after logging out of ChangeBank
Login Request Flow
This is the flow of a user who logs in to both the ChangeBank and ChangeBank Forum applications who checks the Keep me signed in checkbox on the hosted login pages. The refresh token Id is stored in an application session.
Centralized sessions login flow.
Normal Request Flow
This is the flow of a user who has a valid ChangeBank application session and is interacting with the application. FusionAuth is consulted every request.
Centralized sessions normal request flow.
Logout Request Flow
This is the flow of a user who is logging out of ChangeBank.
Centralized sessions logout flow.
Request Flow With Invalid Centralized Session
Suppose a user has logged out of ChangeBank. They have had their refresh tokens revoked and thus their centralized sessions invalidated. But the ChangeBank Forum application still has a valid application session. This flow shows what happens when the user visits ChangeBank Forum.
Centralized sessions with a request when sessions have been invalidated.
Checking Session Validity
With centralized sessions, on each request you must check whether the refresh token associated with the current device is valid. How you do that depends on your application, but one approach is to use middleware.
Here’s code adding middleware to an Express application.
app.use(redirectFunction);
redirectFunction
is defined in a separate file. In this code the refresh token Id is stored as a cookie, but it could be stored in an application session. The code retrieves the Id of the refresh token and checks the validity. The check happens on every request, but you can ignore certain URLs, either by a full path or by path prefix.
const alwaysAllowed = [ "/logout", "/login", "/favicon.ico", "/endsession","/" ];
const alwaysAllowedPrefix = [ "/oauth-redirect", "/static" ];
const {FusionAuthClient} = require('@fusionauth/typescript-client');
const dotenv = require('dotenv');
dotenv.config();
const apiKey = process.env.apiKey;
const fusionAuthURL = process.env.fusionAuthURL;
const client = new FusionAuthClient(apiKey, fusionAuthURL);
const refreshToken = 'refreshTokenCBF';
/**
* Redirect function
* @param {import('express').Request} req - The request object
* @param {import('express').Response} res - The response object
* @param {import('express').NextFunction} next - The next middleware function
*/
function redirectFunction(req, res, next) {
// console.log('url:', req.originalUrl);
if (alwaysAllowed.indexOf(req.originalUrl) > -1 ) {
// always allow this
next();
return;
}
for (let i = 0; i < alwaysAllowedPrefix.length; i++) {
let prefix = alwaysAllowedPrefix[i];
if (req.originalUrl.startsWith(prefix)) {
// always allow this
next();
return;
}
}
const refreshTokenId = req.cookies[refreshToken];
if (!refreshTokenId) {
// console.log("no refresh token");
res.redirect(302, "/login");
return;
}
client.retrieveRefreshTokenById(refreshTokenId)
.then(clientResponse => {
// console.log("valid session found");
next();
return;
}).catch(clientResponse => {
if (clientResponse.statusCode !== 200) {
// console.log("session revoked");
res.redirect(302, "/logout");
next();
return;
}
});
}
module.exports = { redirectFunction };
Using the API to check refresh token validity may not work, depending on your session lifetime preferences.
If you want a rolling window of session validity, use the refresh token grant instead. This will extend the lifetime of the refresh token. It will also trigger a webhook if configured, which can be useful for analytics. You can discard the resulting JWT.
Flexible Revocation
One of the strengths of centralized sessions is custom session invalidation logic. You can write code to control what refresh tokens are revoked when a logout request is processed.
Let’s look at a scenario where custom session invalidation logic would be helpful. Consider these business requirements:
- When a user logs out of ChangeBank, they are also logged out of the ChangeBank Forum.
- When a user logs out of ChangeBank Forum, they are not logged out of ChangeBank. Just because someone doesn’t want to talk about nickels and dimes doesn’t mean they should be logged out of their main application.
- If a user logs out of ChangeBank on one device, they must be logged out from all their devices.
- If a user logs out of ChangeBank Forum, it only affects that particular device.
Here’s example typescript code for the ChangeBank logout route, where all tokens for the user are revoked across all devices.
app.get('/endsession', async (req, res, next) => {
console.log('Ending session...')
const refreshTokenId = req.cookies[refreshToken];
if (refreshTokenId) {
try {
const refreshTokenResponse = await client.retrieveRefreshTokenById(refreshTokenId);
const responseJSON = refreshTokenResponse.response;
if (responseJSON['refreshToken'] && responseJSON['refreshToken']['userId']) {
const userId = responseJSON['refreshToken']['userId'];
await client.revokeRefreshTokensByUserId(userId);
}
} catch(err) {
console.log("in error");
console.error(JSON.stringify(err));
}
}
res.clearCookie(refreshToken);
res.clearCookie(userSession);
res.clearCookie(userToken);
res.clearCookie(userDetails);
res.redirect(302, '/')
});
Here’s example code for the ChangeBank Forum logout route. Here only the refresh token whose Id was previously stored is revoked.
app.get('/endsession', async (req, res, next) => {
console.log('Ending session...')
const refreshTokenId = req.cookies[refreshToken];
if (refreshTokenId) {
try {
await client.revokeRefreshTokenById(refreshTokenId);
} catch(err) {
console.log("in error");
console.error(JSON.stringify(err));
}
}
res.clearCookie(refreshToken);
res.clearCookie(userSession);
res.clearCookie(userToken);
res.clearCookie(userDetails);
// redirect back to changebank
res.redirect(302, 'http://'+cbhostname+':'+cbport+'/account')
});
It’s not just the complex single user logout use case shown above that is supported. Since you can revoke refresh tokens from other applications using the SDKs and APIs, you can have custom session expiration logic.
Examples include:
- When a suspicious login occurs, you can revoke the refresh tokens for the affected user, and force them to re-authenticate. You can also force them to MFA using step up auth.
- Revoke the refresh tokens for all users in a group or with a custom user data value if any single user logs out.
- Enforce a schedule, and revoke access for users every Friday night, forcing them to log in weekly.
- When building or augmenting a customer service application, you can add a button to ‘log the user out’ in the user details screen. In fact, the FusionAuth admin UI provides this functionality. Here’s a screenshot:
All of these are possible because your applications check in with FusionAuth, and FusionAuth provides programmatic control of the centralized sessions.
Using The Login API
If you don’t want to use the hosted login pages, but instead want to create your own user interface, use the Login API. You can create refresh tokens and use centralized sessions when users log in with the Login API.
To obtain refresh tokens, configure the Application to allow refresh tokens using the Login API. Navigate to Applications -> Your Application -> Security . Make sure the Application has the Enable JWT refresh checkbox toggled. The Generate refresh tokens setting must be enabled too.
Build the same refresh token validity check and revocation logic into the logout functionality of your application as shown above.
Timeouts And Session Lifetimes
A centralized session can be created only by using the Login API, when configured as documented in Using The Login API, or by completing the OAuth Authorization Code grant with the offline_access
scope requested. There is no API for creating a centralized session, though there is an open GitHub issue.
A centralized session will end when:
- It expires.
- It is deleted using the API or a corresponding SDK call.
- Optionally, as a result of a user changing their password or having their account locked.
The timeout of refresh tokens are controlled at the Tenant level, under Tenants -> Your Tenant -> JWT . In the Refresh token settings, there is a Duration field. Durations have a unit of minutes. The minimum lifetime of a centralized session is one minute. Timeouts can be overridden at the Application level if different web or mobile applications need different session durations.
The lifetime is controlled by the Tenant or Application refresh token expiration policy as well. Options include:
- have a fixed lifetime
- a lifetime which resets each time the refresh token is used
- a lifetime which resets on use up to a maximum duration
Please review the Tenant API for more information about the policy and its options.
Ensure your application session timeout is longer than the timeout of the centralized session so you don’t inadvertently log someone out before their centralized session expires.
Testing Logins
If a user is repeatedly logging in and creating a refresh token, they should log out or revoke the tokens periodically. This behavior often happens in automated testing.
The list of tokens is visible in the admin UI. Here’s an example:
If the list of sessions is long in a production or QA context, you may not be appropriately revoking refresh tokens.
But Aren’t Refresh Tokens Used For Minting JWTs?
Yes. Yes they are.
But for FusionAuth, refresh tokens serve two purposes.
- Refresh tokens represent a user/device pair as a session.
- Refresh tokens can be used as RFC 6749 compatible refresh tokens for creating access tokens using the Refresh grant. These access tokens would then be presented to your APIs or servers for access.
JWTs And Sessions
You can use the JWTs generated by the authentication process as a distributed session for other applications. This is common practice with APIs and single-page applications (SPAs). This is an example of decentralized API key authentication.
JWTs represent a session to other services, but the refresh token represents the session within FusionAuth.
When you use JWTs in this way, you are making a tradeoff:
The JWT represents a decentralized session which can be stored by the client after authentication and presented to other services as proof that the user has authenticated. It is decentralized because a JWT can be verified without consulting FusionAuth. This means that FusionAuth isn’t consulted when a service receives a JWT to determine if it is valid, which lowers the availability and performance requirements of FusionAuth.
On the other hand, because the JWT is decentralized, revocation of the session becomes difficult. There are ways to offer JWT revocation, but they can be cumbersome. Revoking the refresh token eventually prevents access using a JWT, after the JWT expires.
Working With The FusionAuth SSO Session
If you are using the FusionAuth SSO session as well as centralized sessions, you can delete it in two ways:
- deleting all the refresh tokens associated with a user
- redirecting the users the user to the Front-Channel Logout endpoint
In the latter case, you’ll still need to revoke refresh tokens using the API, because the Front-Channel Logout does not revoke any other refresh tokens.
Sample Project
Here’s an example application showing how to use centralized sessions. While this example focuses on two web apps, the centralized sessions approach is well suited to mobile apps, APIs, or non-browser based applications as well.
Application Managed Sessions
Application managed sessions, the other main approach for session management in FusionAuth, are simpler. However, they don’t offer centralized control of your users’ sessions. Instead, with this approach, you delegate session management to each application.
Implementation Steps
Let’s discuss this in the context of the ChangeBank and ChangeBank Forum applications. To set up application managed sessions for these application:
- Write code which can log a user out when it receives a
GET
request. This code should destroy the application session. It should be idempotent because it may receive more than one request. - Set the Logout URL for each application. This URL should point to the endpoint where the code you wrote is hosted. This value is configured under Applications -> Your Application -> OAuth .
- Configure each application’s Logout behavior to be either All applications or Redirect only. This option controls the behavior when the user logs out. The former option logs the user out of all applications in a tenant by making a request to each application’s configured Logout URL . The latter logs the user out of the single application for which the logout request was made.
- When a user logs out of either the ChangeBank or the ChangeBank Forum application, redirect the user’s browser to the Front-channel Logout endpoint.
That’s it.
With application managed sessions, users can be logged out of multiple applications, though the approach is less granular than that offered by centralized sessions. The Front-channel Logout endpoint will attempt to log the user out based on the Logout behavior value.
If you are using application managed sessions and a user logs out of ChangeBank, but you don’t route them through the Front-channel Logout endpoint, all other applications will be unaffected.
In addition, if the user has a FusionAuth SSO session, the next time the user tries to log in, they’ll be sent to FusionAuth which will transparently log them in, since they were never logged out of the FusionAuth SSO session.
If you want to review application managed sessions in more detail, the single sign-on guide walks you through building an example application.
Let’s look at some example flows of a user logging into the ChangeBank and ChangeBank Forum applications.
Flow Diagrams Of Common Use Cases
Let’s look at some flow diagrams for these four use cases:
- a user logs into ChangeBank and then visits ChangeBank Forum
- a logged in user interacts with ChangeBank
- a user logs out of ChangeBank, which revokes all refresh tokens for that user
- tries to access ChangeBank Forum after logging out of ChangeBank
In these diagrams, the ChangeBank application is configured to log users out of all applications on logout. That is, Logout behavior is All applications.
Login Request Flow
This is the flow of a user who logs in to both the ChangeBank and ChangeBank Forum applications who checks the Keep me signed in checkbox on the hosted login pages.
Application managed sessions login flow.
If you don’t have the Keep me signed in checked, then the user will be prompted to authenticate every time they are sent to FusionAuth. There will be no FusionAuth SSO session.
Normal Request Flow
This is the flow of a user who has a valid ChangeBank application session and is interacting with the application. FusionAuth receives no requests.
Application managed sessions normal requests flow.
Request Flow With Invalid Application Session
This is the flow when an application session expires. The user is then sent to FusionAuth. However, FusionAuth still has a valid SSO session, so the user is logged in without interaction.
Application managed sessions when the application session is invalid, but the SSO session is still valid.
Logout Request Flow
This is the flow of a user who is logging out of ChangeBank.
Application managed sessions logout flow.
Timeouts And Session Lifetimes
With this approach, each application manages session timeouts, including idle timeouts.
FusionAuth has no information about each application session duration, current status, or devices attached.
Redirecting Users On Logout
Adding a post_logout_redirect_uri
parameter to the Front-Channel Logout endpoint request allows you to send different users to different logout pages.
Each URL that might be added as a value must be included in the Application’s Authorized redirect URLs list. Learn more about adding URLs to that list..
Let’s look at a scenario where this would be useful. Suppose you have three tiers of users in the ChangeBank application:
- Enterprise
- Premium
- Free
After a user has logged out, you need to send them to a different page based on their tier. Create these URLs, make sure they display different messages, and register them as authorized redirect URLs.
https://example.com/logout/thank-you-so-much
for the enterprise customers, where you thank them profusely for using your software.https://example.com/logout/thanks
for the premium customers, where you thank them.https://example.com/logout/consider-paying-us
for the free tier customers, where you thank them but also try to upsell them.
Then, in the code which generates the logout URL, you add the correct value as a post_logout_redirect_uri
. Make sure you escape the URL. The user will then be sent to the appropriate thank you page.
For example, suppose FusionAuth is running at https://auth.example.com
, the ChangeBank application has a client Id of e9fdb985-9173-4e01-9d73-ac2d60d1dc8e
, and the user is a premium user. The logout URL would be: https://auth.example.com/oauth2/logout?client_id=e9fdb985-9173-4e01-9d73-ac2d60d1dc8e&post_logout_redirect_uri=https%3A%2F%2Fexample.com%2Flogout%2Fthanks
.
Working With Centralized Sessions
The code at the Logout URL which has to terminate the application session can also make API calls and revoke FusionAuth refresh tokens. Doing so lets you combine this approach with centralized sessions.
Sample Project
Here’s two example web apps with application managed sessions.
FusionAuth SSO
In Types of Sessions, you learned about the FusionAuth SSO Session. This section will discuss it in more depth. The FusionAuth SSO session is managed by FusionAuth.
This session can be bootstrapped from an access token if you are using the Login APIs, but is more typically used with the hosted login pages. The rest of this doc, except the Bootstrapping SSO section, assumes you are using the FusionAuth SSO session with the hosted login pages.
You can use FusionAuth with or without the FusionAuth SSO session. If you do not use this session, users must authenticate every time their application session expires or if they switch to a different application.
To enable the SSO session, set the rememberDevice
parameter on the login page to true
. This is the Keep me signed in checkbox in the default theme. This value can be set by an end user checking a checkbox or it can be a hidden field in the login form.
To customize the lifetime for this session, navigate to Tenants -> Your Tenant -> OAuth . The Session Timeout must be a positive integer. The unit is seconds. Since the FusionAuth SSO session lets users log into every application in a tenant, there is no application level override of the SSO session duration.
In this context, SSO or single sign-on, is the ability of a user to switch between different applications without authenticating each time. It is accomplished by delegating authentication to FusionAuth. Here’s a guide walking through this functionality in more detail.
FusionAuth SSO is not the same concept as a single sign-on to Google, Facebook, or another external identity provider. Such functionality, also called federation, is handled in FusionAuth by Identity Providers.
When the rememberDevice
value is true
, FusionAuth creates a session for the user within FusionAuth. When the same device visits the hosted login pages and has a valid SSO session, the user is transparently logged in. They’ll be sent to the requested application redirect URL with an authorization code. This code can be exchanged for a token.
This transparent authentication flow follows the same process and rules as any other authentication in FusionAuth. These include but are not limited to:
- If an application requires the user to be registered and they are not, they’ll be presented with an error screen.
- If an application requires email verification and the user has not completed such a verification, they’ll be prompted to do so.
Only if all the authentication conditions are met will the authentication be truly transparent.
Session Timeouts and Lifetime
The FusionAuth SSO session allows transparent authentication on one browser or device until one of the following happens:
- the SSO session expires
- the user is logged out by being sent to the Front-Channel logout endpoint
- the refresh token representing the FusionAuth SSO session is revoked via an API call or the admin UI
Previous to version 1.52, setting the SSO session to a low value and enabling other post authentication workflows such as an OAuth consent screen could cause a login workflow to be restarted. See the release notes for version 1.53.0 for more details.
The single sign-on session duration can be configured at the Tenant level. Navigate to Tenant -> Your Tenant -> OAuth and edit the Session timeout value. Because this value is shared between applications, it can’t be overridden in application configuration.
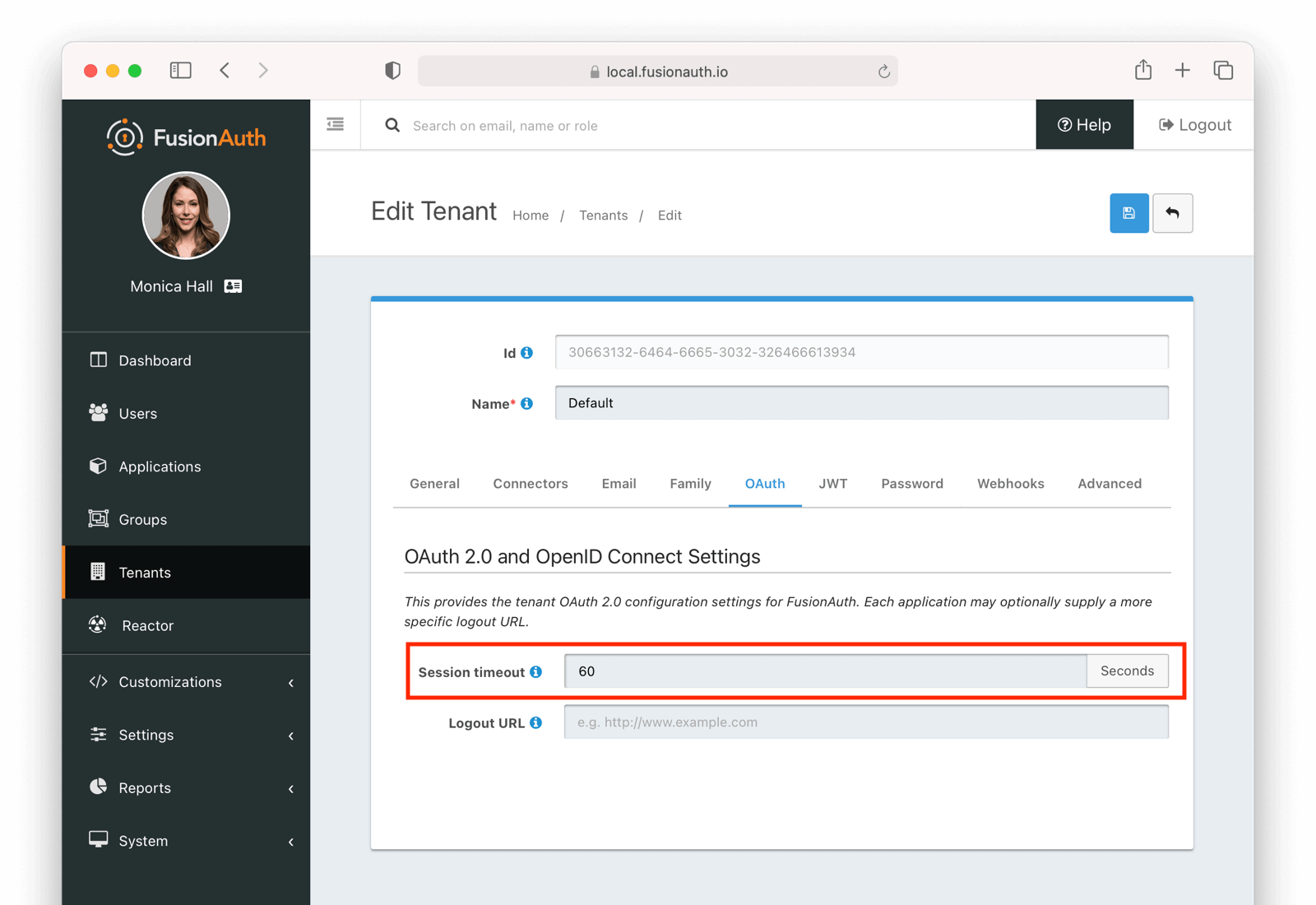
The length of a single sign-on session can be different from the session length for individual applications. When a request to an application occurs, there are four possible scenarios:
Single sign-on session scenarios
Application Session | FusionAuth Session | Result |
---|---|---|
valid | valid | Application serves request. |
valid | expired | Application serves request. |
expired | valid | Application redirects to FusionAuth, which redirects back to the application. The application then adds the user to the session and serves the request. |
expired | expired | The user must authenticate with FusionAuth. On successful authentication, FusionAuth redirects back to the application. The application then adds the user to the session and serves the request. |
Disabling FusionAuth SSO
If you don’t want FusionAuth SSO to be enabled, set the rememberDevice
parameter to false
in the login page. In this case, there will be no SSO session. You can also set the Session Timeout to zero.
You can selectively disable the FusionAuth SSO session. For example, suppose you have five applications which delegate authentication to FusionAuth. If four of the applications are consumer facing, but the fifth is an internal business application, you might want to let users transparently log in between the consumer facing apps, but not the business app.
Options to implement this behavior include:
- Place the business application in a separate FusionAuth tenant. Now your users need to manage two separate logins and there might be credential drift. You can work around this by having users who need access to both the business application and the consumer facing applications use the same Identity Provider to log in across tenants.
- Require registration for the business application and turn off self-service registration for that application. Then you’ll need to add a registration for all users who need access to the business application manually or using the API.
Bootstrapping SSO
In certain scenarios you may have authenticated a user outside of the typical OAuth flow. If so, you can still acquire an SSO session from FusionAuth as long as you have a valid access token for the user.
There are one-time setup steps:
- Enable the access token bootstrap setting on the tenant either via the Allow access token bootstrap setting in the Admin UI or by setting the tenant.ssoConfiguration.allowAccessTokenBootstrap field to
true
in the Tenant API. - FusionAuth requires the access token to be in an
Authorization
header. Because browsers do not provide a way to set theAuthorization
header when browsing to a location, you’ll need to add the header using, for example, a reverse proxy.
To set up the SSO session for a given user:
- provide the access token as a
Bearer
token in theAuthorization
header - redirect the user’s browser to the
/oauth2/authorize
endpoint, including aredirect_uri
parameter
The header will need to be in the following form:
Authorization: Bearer <access token>
If the token is valid, FusionAuth will create an SSO session and redirect the browser to the provided redirect_uri
. This redirect_uri
parameter must be an authorized URI, registered with the given application. See the OAuth Authorize documentation for details.
Session Analytics
For application managed sessions, there are minimal analytics available, as FusionAuth only captures information about the login. This is available via the Search Login Records API.
FusionAuth has prebuilt session visibility when using centralized session management. View current sessions for a user by navigating to Users -> A User in the administrative user interface. Then look at the Sessions tab.
If you need more in-depth insights into sessions, set up webhooks for:
- User login, sent when a session is created.
- Refresh Token use, sent when a session is extended.
- Refresh Token revocation, sent when a session is revoked.
These events can be stored and correlated based on the user Id to generate statistics around the average duration of a session, data attributes, or number of sessions.
Other Ways To Logout
While refresh token revocation, calling the Logout URL and using the Front-Channel Logout are the main ways of logging a user out of FusionAuth, there are some other options too.
SAML Single Logout
If you are using FusionAuth as a SAML IdP you can also enable SAML Single Logout. FusionAuth will then work with off the shelf commercial applications which support the SAMLv2 Single Logout profile.
Learn more about configuring SAML Single Logout here.
Logging Out Of Identity Providers
FusionAuth does not support logging the user out of Identity Providers. In other words, if someone logs in using a Google Identity Provider, then logs out of FusionAuth, they won’t be logged out of Google.
This is typically the behavior you want.
If you want users logged out of the Identity Provider, a workaround, if the Identity Provider has a well known logout endpoint, is to add that as a post_logout_redirect_uri
and send the user’s browser there after they’ve logged out of FusionAuth.
This only works if you know the user logged in with that Identity Provider, which is currently available on the login success webhook. So you’d have to capture that to build the correct post_logout_redirect_uri
.
Logging Out Of The Account Application
If you have enabled the self-service account management functionality of FusionAuth, this has a separate session from the FusionAuth SSO session or your application session.
You must use the account logout endpoint to end this session.
To do so, redirect the user to /account/logout?client_id=...
where the client_id
is the application Id for which you have set up self-service account management.
This behavior is explained further in this GitHub issue.
The /api/logout
Endpoint
You can use the /api/logout
endpoint in certain circumstances.
This is designed for situations where you store the refresh token in a cookie and want to revoke it from the client without an API key.
Using this endpoint is uncommon.
Other Resources
- The Device Limiting guide discusses sessions as well.
- The Applications Core Concepts covers many of these settings.
- The Front-Channel Logout Endpoint documents this form of logout.
- The Single Sign-on guide discusses FusionAuth SSO session usage in detail.